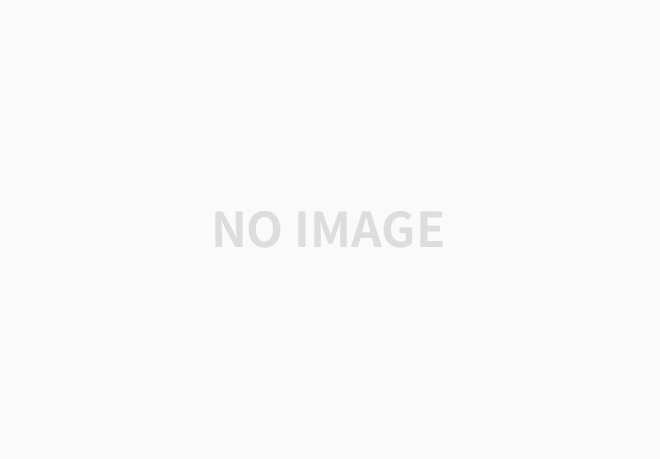
이번 시간에는 Go언어의 코드 분석과
Go를 이용해 웹 페이지를 띄우는 방법을
알아보도록 하겠습니다.
Go 참고 문서
The Go Programming Language
DevOps & Site Reliability With fast build times, lean syntax, an automatic formatter and doc generator, Go is built to support both DevOps and SRE.
go.dev
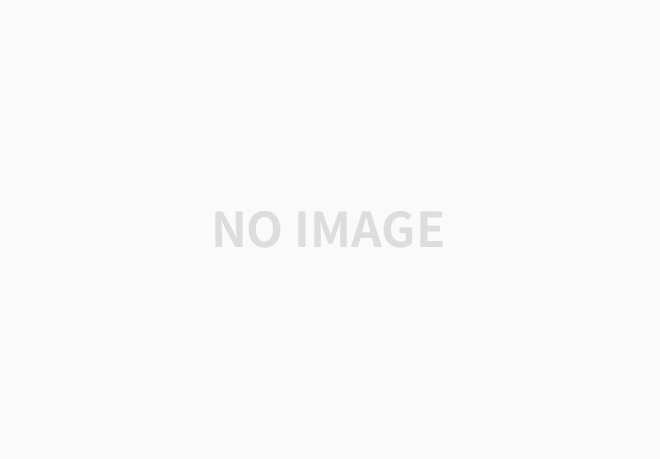
위 사이트로 들어가면 Go언어를
직접 출력할 수 있는 도구가
있습니다.
해당 도구로 Go언어를 설치하지
않고도 직접 사이트에서
코드를 입력해 출력할 수 있습니다.
Standard library - Go Packages
Directories ¶ Expand all tar Package tar implements access to tar archives. Package tar implements access to tar archives. zip Package zip provides support for reading and writing ZIP archives. Package zip provides support for reading and writing ZIP arch
pkg.go.dev
Go Packages에서는
Go의 기본 라이브러리에 관한
문서를 제공하고 있습니다.
또한 소스 코드도 함께 제공하므로
문서만으로 동작 방식을 이해하기 어렵다면
위 사이트를 통해 직접 확인할 수 있습니다.
Go Playground - The Go Programming Language
// You can edit this code! // Click here and start typing. package main import "fmt" func main() { fmt.Println("Hello, 世界") } About the Playground The Go Playground is a web service that runs on go.dev's servers. The service receives a Go program, vets
go.dev
Go Playground 사이트에서도
Go 코드를 직접 컴파일 하고 실행할 수 있습니다.
간단한 문법을 확인해 보거나 실행 결과를
살펴볼 때 유용하고
웹 페이지에서 코드를 작성 후
Run 버튼을 클릭해 실행 결과 화면을
직접 보여줍니다.
Go Packages
pkg.go.dev
Go의 기본 라이브러리가 아닌
커스텀 패키지에 관한 문서는
GoDoc 웹 페이지에
게시되어 있습니다.
Godoc 명령어
godoc 명령어를 입력하면
Go 공식 문서를 로컬 웹 서버에서
볼 수 있습니다
koras02@jung-desktop ~/study/go/go-blog > godoc -http=:8000
using GOPATH mode
cmd 창에 위 명령어를 입력 해 실행한 후
웹 브라우저에 http://localhost:8000/로
접속하면 Go 공식 문서를 로컬 웹 서버에서
볼수 있습니다. godoc 명령어를 로컬 웹 서버로
실행하면 현재 PC에 받았던 외부 패키지에
대한 문서도 함께 보여줍니다.
koras02@jung-desktop ~/study/go/go-blog> main > go doc fmt.Printf
package fmt // import "fmt"
func Printf(format string, a ...interface{}) (n int, err error)
Printf formats according to a format specifier and writes to standard
output. It returns the number of bytes written and any write error
encountered.
go doc image/png를 입력 후
실행하면 image/png 패키지 전체 정보를
명령 프롬프트에서 보여 줍니다.
koras02@jung-desktop ~/study/go/go-blog ↱ main godoc image/png
Unexpected arguments. Use "go doc" for command-line help output instead. For example, "go doc fmt.Printf".
usage: godoc -http=localhost:6060
-analysis string
comma-separated list of analyses to perform when in GOPATH mode (supported: type, pointer). See https://golang.org/lib/godoc/analysis/help.html
-goroot string
Go root directory (default "/usr/lib/go-1.13")
-http string
HTTP service address (default "localhost:6060")
-index
enable search index
-index_files string
glob pattern specifying index files; if not empty, the index is read from these files in sorted order
-index_interval duration
interval of indexing; 0 for default (5m), negative to only index once at startup
-index_throttle float
index throttle value; 0.0 = no time allocated, 1.0 = full throttle (default 0.75)
-links
link identifiers to their declarations (default true)
-maxresults int
maximum number of full text search results shown (default 10000)
-notes string
regular expression matching note markers to show (default "BUG")
-play
enable playground
-templates string
load templates/JS/CSS from disk in this directory
-timestamps
show timestamps with directory listings
-url string
print HTML for named URL
-v verbose mode
-write_index
write index to a file; the file name must be specified with -index_files
-zip string
zip file providing the file system to serve; disabled if empty
✘ koras02@jung-desktop ~/study/go/go-blog ↱ main go doc image/png
package png // import "image/png"
Package png implements a PNG image decoder and encoder.
The PNG specification is at https://www.w3.org/TR/PNG/.
func Decode(r io.Reader) (image.Image, error)
func DecodeConfig(r io.Reader) (image.Config, error)
func Encode(w io.Writer, m image.Image) error
type CompressionLevel int
const DefaultCompression CompressionLevel = 0 ...
type Encoder struct{ ... }
type EncoderBuffer encoder
type EncoderBufferPool interface{ ... }
type FormatError string
type UnsupportedError string
Go 기본 문법
Go는 간결하고 명확한 문법에 중접을 두고
설계된 언어입니다.
그래서 코드가 좀더 단수해졌고 가독성이
높아졌습니다.
이번에는 Go의 기본 문법을 알아보도록
하겠습니다.
시작 하기전 지난번 설치 과정에서
실행한 Go 코드를 다시 살펴 봅시다.
// 1.패키지 선언
package main
//2.외부 패키지 import
import "fmt"
// 3.main 함수
func main() {
// 4.외부 패키지 사용
fmt.Println("Hello World")
}
Go 문법의 간결함과 유연함
Go는 문법 요소는 줄이되 유연함을 더욱 높혔습니다.
그렇기에 적은 문법으로 풍부한 기능 구현이
가능하고, Go에서는 while문이 없어
for 반복문으로만 반복을 표현할 수 있습니다.
또한 복잡한 if문 대신 switch문 case에
조건식을 넣어 간결하게 표현할 수 있습니다.
// go - 1.for 반복문 1
package main
import "fmt"
func main() {
sum :=0
// for문에 초기화 구문, 조건식, 후속 작업 순으로 정의
// 실행 결과 45
for i :=0; i < 10; i++ {
sum += i
}
fmt.Println(sum)
}
// go - for 반복문2
package main
import "fmt"
func main() {
sum, i := 0,0
// for 문에 조건식만 사용
// 실행 결과 45
for i < 10 {
sum += i
i++
}
fmt.Println(sum)
}
아래는 switch 함수를 사용한 예 입니다.
// switch 문 case 조건식 사용예
package main
import "fmt"
func main() {
c := 'H'
switch {
// case 에 조건식을 사용
case '0' <= c && c <= '9':
fmt.Printf("%c은(는) 숫자입니다.",c)
case 'a' <= c && c <= 'z':
fmt.Printf("%c은(는) 소문자입니다.", c)
case 'A' <= c && c <= 'Z':
fmt.Printf("%c은(는) 대문자 입니다.", c)
}
}
참고자료
Go 언어 웹 프로그래밍 철저 입문: 1장. Go 시작하기
더북(TheBook): (주)도서출판 길벗에서 제공하는 IT 도서 열람 서비스입니다.
thebook.io
GitHub - Koras02/go-tutorial-blog: https://koras02.tistory.com/category/%ED%94%84%EB%A1%A0%ED%8A%B8%20%EC%97%94%EB%93%9C/Go
https://koras02.tistory.com/category/%ED%94%84%EB%A1%A0%ED%8A%B8%20%EC%97%94%EB%93%9C/Go - GitHub - Koras02/go-tutorial-blog: https://koras02.tistory.com/category/%ED%94%84%EB%A1%A0%ED%8A%B8%20%EC%...
github.com
'프론트 엔드 > Go' 카테고리의 다른 글
[Go] Go 정적 타입 언어, 동적 프로그래밍 세미콜론, 모호한 요소제거, 주석 사용법 (0) | 2023.01.11 |
---|---|
[GO] 리눅스 버전 Go 설치하기 - 헬로우 월드 출력 (0) | 2023.01.09 |
[Go] GO를 이용해 HTTP Server 만들기 (0) | 2022.03.03 |